Building Micro-frontends using Blazor and Piral Framework⚡

Hey everyone 👋 , the main focus of this article is to explain how we can build micro-frontends using Microsoft Blazor and Piral Framework.
Managing large-scale web applications can get super complex as the application grows and scales up. Micro-frontends are a strategic approach to building modular web apps that helps teams work independently to manage large products.

As teams develop and deploy their micro-frontends independently, they can iterate quickly and adapt to changing requirements with agility. Micro-frontends also facilitate continuous integration and deployment, enabling teams to release updates to their components faster without disrupting the entire application.
If you are totally new to the area of Micro-frontends, I would recommend you to first watch this short video by Jack Herrington.
🏗️ Architectural patterns to build micro-frontends.
Micro-Frontends (MFEs) can be implemented using various architectural patterns, each offering different trade-offs in terms of flexibility, complexity, and performance. The two main patterns are Compile-Time Composition and Run-Time Composition.
📌 Pattern 1: Compile-Time Composition
In the compile-time composition pattern, the frontend is composed during the build process, usually at compile time. Each micro frontend is developed as an independent module or package, typically with its own repository. During the build phase, these micro-frontends are integrated together to compose the resulting application which is visible to the end user.

✅ Pros:
- Performance Optimization: Since the integration happens at build time, the resulting application is often more performant with fewer runtime dependencies.
- Simplified Deployment: With everything bundled during the build process, deployment is more straightforward, as there is only one artifact to deploy.
- Consistency: All micro-frontends are bundled together, ensuring consistency in versions of shared dependencies.
❌ Cons:
- Inflexibility: Changing or updating a single micro frontend requires rebuilding and redeploying the entire application.
- Longer Build Times: Integrating multiple micro-frontends during the build can result in longer build times, especially as the application scales.
- Dependency Management: Managing shared dependencies can be complex, especially if different teams use different versions.
- Scalability: As the number of micro-frontends increases, the complexity of the build process can grow, leading to potential maintenance challenges.
📌 Pattern 2: Run-Time Composition
Runtime composition assembles the frontend at runtime, typically in the browser or server-side. Each micro-frontend remains independent and is responsible for rendering its UI and managing its own state. During runtime, a shell application or orchestrator dynamically fetches and integrates the necessary micro-frontends to construct the complete user interface.

✅ Pros:
- Flexibility: Individual micro-frontends can be updated and deployed independently without affecting the rest of the application.
- Scalability: Easier to scale as each micro-frontend can be developed, tested, and deployed separately.
- Dynamic Loading: Only the necessary micro-frontends are loaded at runtime, which can reduce initial load times.
- Simplified Development: Teams can work on different micro-frontends independently, leading to faster development cycles.
❌ Cons:
- Performance Overhead: Run-time composition may introduce additional performance overhead due to the dynamic fetching and integration of micro-frontends.
- Complexity in Orchestration: Managing state and communication between micro-frontends at runtime can be more complex, especially when dealing with multiple teams.
- Versioning Challenges: Ensuring compatibility between different versions of micro-frontends can be tricky, especially in larger applications.
- Security Concerns: Dynamically loading micro-frontends may introduce security risks if not properly managed.
🔥 Quick refresher on Microsoft Blazor
As described in the official documentation, Blazor is a modern front-end web framework based on HTML, CSS, and C# that helps you build web apps faster. With Blazor, we can build web apps using reusable components and it is packed with a lot of rich features. Blazor offers multiple hosting models and the main two models are Server-side and WebAssembly. In the server-side model, the bulk of the processing happens on the server, while in WebAssembly, .NET code runs directly in the browser.
The architecture for micro-frontends proposed in this article is for the Blazor Server-side hosting model. However, it is possible to achieve the same results with WebAssembly model with some modifications to the architecture.
🎯 The Piral Framework
The Piral Framework is an open-source framework for building modular and scalable web applications using a micro-frontend architecture. It allows developers to create and compose multiple independent modules or micro-frontends into a single, cohesive application in run time.

Key features of the Piral Framework include:
- Modularity: Piral enables developers to break down their application into smaller, self-contained modules called “pilets.” Each pilet can encapsulate its own logic, UI components, styles, and dependencies, allowing for better organization and maintainability of code.
- Dynamic Composition: Piral provides mechanisms for dynamically composing and integrating pilets into the application at runtime. This allows for flexibility in adding, removing, or updating features without disrupting the entire application.
- Extensibility: Developers can extend the functionality of their Piral application by incorporating existing pilets from a shared repository or by creating custom pilets tailored to their specific needs. This promotes reusability and collaboration across projects.
- Developer Experience: Piral offers a streamlined developer experience with features like hot module replacement (HMR), automatic dependency resolution, and a CLI (Command Line Interface) for scaffolding and managing projects.
🚀 Proposed Architecture
Now, let’s dive deeper into how we can implement micro-frontends using Blazor and the Piral framework. The proposed architecture focuses specifically on the Blazor Server-side hosting model and run-time composition is used to dynamically assemble the final application. The diagram below provides a high-level overview of this architecture, highlighting the key components and their interactions.

The architecture comprises of a Blazor server app shell project and several micro-frontend projects, designed to work together. The app shell is equipped with an orchestrator that handles the dynamic run-time composition of micro-frontends, which communicates with Piral Cloud to fetch and integrate these components. This enables greater flexibility in deployment and updates, as individual micro-frontends can be modified or replaced without requiring a full rebuild of the entire application. In other words, each micro-frontend can be developed, maintained, and versioned independently, with separate repositories allowing different teams to manage them as shown in the above diagram.
The micro-frontends are built into NuGet packages and published to Piral Cloud, and this process that can be automated through a CI/CD pipeline (Eg: Github actions) to ensure consistent and timely updates. Piral Cloud also manages versioning and provides an admin dashboard where you can enable or disable micro-frontends, configure rules, and toggle features.
The app shell is designed with slots that can accommodate both large micro-apps and smaller components, allowing for dynamic and efficient integration at runtime. This modular setup ensures that while the underlying system is complex, end users experience a unified application, with the micro-frontends blending into a smooth user experience.
🚧 Implementation and Starter project
In this section, we will explore the implementation of the architecture outlined previously by breaking down the steps needed to get the starter project up and running while focusing on integrating with the Piral cloud.
1. Set up a Piral Cloud account and configure a feed
Head over to https://portal.piral.cloud/ and sign up for a new account if you don’t already have one. Once logged in, you’ll be able to create a new feed, as shown below.

That’s all you need to do in Piral Cloud for now. You’ll use the feed name you created later in the process when configuring the discovery of micro-frontends from the AppShell. This feed name will help the App Shell locate and integrate the micro-frontends dynamically during runtime.
Note: Piral cloud also offers are docker image, that can be use to host a instance of the Piral cloud in your own infrastructure if you have any security and privacy concerns.
2. Clone starter project
The starter template linked below serves as a foundational starting point for building modular web applications using the Blazor combined with the Piral.
Clone the repository to you local machine and open up the project in you preferred IDE.
git clone https://github.com/wdevon99/Blazor-Microfrontends.git
The solution should contain the AppShell project and two sample micro-frontend projects as shown in the screenshot below.

3. Configure and run app shell
In the appsettings.json file of the BlazorApp.Shell project, replace the <<FEED_NAME>> with the name of the feed you have created in step 1.
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*",
"Microfrontends": {
"CacheDir": ".cache",
"DiscoveryInfoUrl": "https://feed.piral.cloud/api/v1/microfrontends/<<FEED_NAME>>",
"DiscoveryUpdateUrl": "wss://feed.piral.cloud/api/v1/pilet/<<FEED_NAME>>",
"NugetFeeds": {
"Source": {
"Url": "https://feed.piral.cloud/api/v1/nuget/<<FEED_NAME>>/index.json"
},
"Public": {
"Url": "https://api.nuget.org/v3/index.json"
}
}
}
}
Once you run the BlazorApp.Shell project after adding the feed name, the application should start up in http://localhost:7012 and display an empty dashboard as seen below. (This is empty because we have not yet published the micro-frontends to the Piral cloud feed).

If you have a look at the Dashboard.razor in the Pages folder in BlazorApp.Shell project, you will be able to observe the slots that are placed to enable the micro-frontends to be rendered in.
@page "/"
@using Piral.Blazor.Shared
<PageTitle>Dashboard</PageTitle>
<div class="container">
.......
.......
.......
<MfComponent Name="microfrontend.one" />
<MfComponent Name="microfrontend.two" />
</div>
If you want to create the app shell from scratch, start by setting up a new Blazor Server project and follow the steps mentioned in the following documentation: https://blazor.piral.io/getting-started/server/setup.
4. Publishing the Micro-frontends to Piral Cloud
In order for the App Shell to be able to discover and compose the micro-frontends, we should build and publish them to Piral cloud.
In the sample starter project, there are two Micro-frontend projects:
- Micro-frontend one: The first micro-frontend (Microfrontend.one) is a very simple counter application as shown below.

2. Micro-frontend two: The second micro-frontend is a web application that can show the weather conditions of a given set of cities. It calls the Open Weather Map (api.openweathermap.org) API to fetch weather data. (You have to add you own API key for it to be working as expected) 😃.

If you want to create the micro-frontend projects from scratch, start by create a new Razor class library project and follow the steps mentioned in the following documentation: https://blazor.piral.io/getting-started/server/microfrontend.
To publish the micro-frontends, we can use the piral-server-server CLI. You will need a key in order to authenticate and successfully publish the micro-frontend package and you can get this key from Piral cloud. The publish command is mentioned below.
piral-blazor-server publish-microfrontend --source ./SomeMf --url https://feed.piral.cloud/api/v1/nuget/myfeed/index.json --key abcdef1234
More detailed steps and alternative ways of publishing can be found in the following documentation: https://blazor.piral.io/getting-started/server/publishing
Once you publish the micro-frontends, it should appear in Piral cloud as shown in the below screenshot.

You can click on it and view more details such as the version number, published date and some insights as shown below.

When you run the App Shell now and open it up in you browser, it should show both the micro-frontends rendered in to the slots as shown in the screenshot below.

If you disable a micro-frontend, it would not appear. As demostated below, Micro-frontend one disabled and then only micro-frontend two is rendered in the app shell.

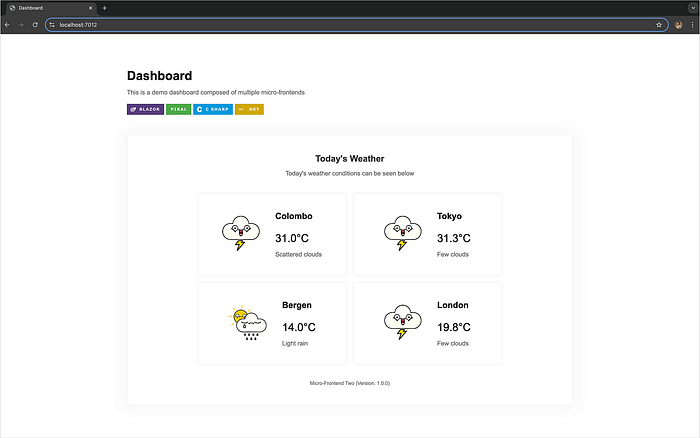
5. Hosting application
To host the application, the app shell should be deployed to your preferred cloud provider with the appropriate configurations. Ensure that the hosting environment is set up to support Blazor Server and the configs for interacting with Piral Cloud. Proper configurations will ensure that the app shell can dynamically load and integrate micro-frontends at runtime.
🏁 Conclusion
As explained in this blog, implementing micro-frontends using Blazor and the Piral framework offers a powerful and flexible approach to managing large-scale web applications. By adopting a modular architecture with run-time composition, you can achieve greater scalability, maintainability, and agility in your development process. The step-by-step guide and sample project provided in this post should give you a solid foundation to start building your own micro-frontend architecture.
As you explore this approach further, you’ll find that the combination of Blazor’s robust component model and Piral’s dynamic composition capabilities can significantly enhance your ability to deliver modular, independent, and feature-rich web applications. Embrace these tools, experiment with the architecture, and unlock new possibilities for your projects.
Special thanks to Florian Rappl and Hasith Yaggahavita for the support given while exploring micro-frontend architectures!
For further clarifications or assistance, feel free to reach out via wdevon99@gmail.com or connect with me through LinkedIn, https://www.linkedin.com/in/devon-wijesinghe. Cheers! 🤜🤛